채팅프로그램 만들기는 총 12개의 게시글로 구성되어있습니다.
두번째 게시글 : 1:1지속성통신(동기서버 완성본 동기 클라이언트)
네번째 게시글: 1:N통신(여기서부터는 여러명을 받아야하므로 당연히 비동기서버입니다.)
일곱번째 게시글 : wpf를 통해 View를 구현한 서버
여덟번째 게시글 : wpf를 통해 View를 구현한 클라이언트(메인화면 만들기)
아홉번째 게시글 : wpf를 통해 View를 구현한 클라이언트(로그인화면 만들기)
열번째 게시글 : wpf를 통해 View를 구현한 클라이언트(채팅상대 선택화면 만들기)
열한번째 게시글 : wpf를 통해 View를 구현한 클라이언트(채팅화면 만들기)
열두번째 게시글 : wpf를 통해 View를 구현한 클라이언트(로직 구현)
이번게시글에서는 채팅화면을 만들겠습니다.
채팅화면은 서버에서 열어줘야하지만
테스트를 위해 직접 열어서 입력하는 방식을 사용하겠습니다.
해당 파일의 시작점을 변경하여 테스트하였습니다.
1. App.xaml
<Application x:Class="ChattingClient.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:ChattingClient"
StartupUri="ChattingWindow.xaml">
<Application.Resources>
</Application.Resources>
</Application>
윈도우앱의 시작파일을 바꾸고싶다면 StartupUri를 변경해 주면 됩니다.
MainWindow.xaml -> ChattingWindow.xaml로 변경해줍니다.
2. ChattingWindow.xaml
<Window x:Class="ChattingClient.ChattingWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
PreviewKeyDown="Window_PreviewKeyDown"
xmlns:local="clr-namespace:ChattingClient"
mc:Ignorable="d"
Title="ChattingWindow" Height="500" Width="500" Background="#BCBCBC">
<Grid Margin="8">
<Grid.RowDefinitions>
<RowDefinition Height="6*"/>
<RowDefinition Height="1*"/>
</Grid.RowDefinitions>
<ListView x:Name="messageListView" Grid.Row="0" Margin="0,0,0,10">
</ListView>
<Grid Grid.Row="1">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="4*"></ColumnDefinition>
<ColumnDefinition Width="1*"></ColumnDefinition>
</Grid.ColumnDefinitions>
<TextBox Grid.Column="0" x:Name="Send_Text_Box"></TextBox>
<Button Grid.Column="1" x:Name="Send_btn" Margin="10,0,0,0" Content="전송" Click="Send_btn_Click"></Button>
</Grid>
</Grid>
</Window>
채팅을 할때는 엔터키를 입력해야하므로
Window태그안에 PreviewKeyDown = "Window_PreviewKeyDown" 이라는 코드를 추가해줍니다.
3. ChattingWindow.cs
namespace ChattingClient
{
/// <summary>
/// ChattingWindow.xaml에 대한 상호 작용 논리
/// </summary>
public partial class ChattingWindow : Window
{
private ObservableCollection<string> messageList = new ObservableCollection<string>();
private string chattingPartner = "";
public ChattingWindow()
{
InitializeComponent();
chattingPartner = "테스트";
messageListView.ItemsSource = messageList;
this.Title = chattingPartner + "님과의 채팅방";
}
private void Send_btn_Click(object sender, RoutedEventArgs e)
{
if (string.IsNullOrEmpty(Send_Text_Box.Text))
return;
string message = Send_Text_Box.Text;
if (chattingPartner != null)
{
messageList.Add("나: " + message);
Send_Text_Box.Clear();
}
if (VisualTreeHelper.GetChildrenCount(messageListView) > 0)
{
Border border = (Border)VisualTreeHelper.GetChild(messageListView, 0);
ScrollViewer scrollViewer = (ScrollViewer)VisualTreeHelper.GetChild(border, 0);
scrollViewer.ScrollToBottom();
}
}
private void Window_PreviewKeyDown(object sender, KeyEventArgs e)
{
if (e.Key == Key.Enter)
{
if (string.IsNullOrEmpty(Send_Text_Box.Text))
return;
string message = Send_Text_Box.Text;
if (chattingPartner != null)
{
messageList.Add("나: " + message);
Send_Text_Box.Clear();
}
if (VisualTreeHelper.GetChildrenCount(messageListView) > 0)
{
Border border = (Border)VisualTreeHelper.GetChild(messageListView, 0);
ScrollViewer scrollViewer = (ScrollViewer)VisualTreeHelper.GetChild(border, 0);
scrollViewer.ScrollToBottom();
}
}
}
}
}
전송하기버튼과 엔터키 입력의 기능을 구현합니다.
ListView의 아이템이 ListView의 높이보다 길어졌을때
스크롤을 최하단으로 움직여주는 코드를 추가합니다.
4. 실행화면
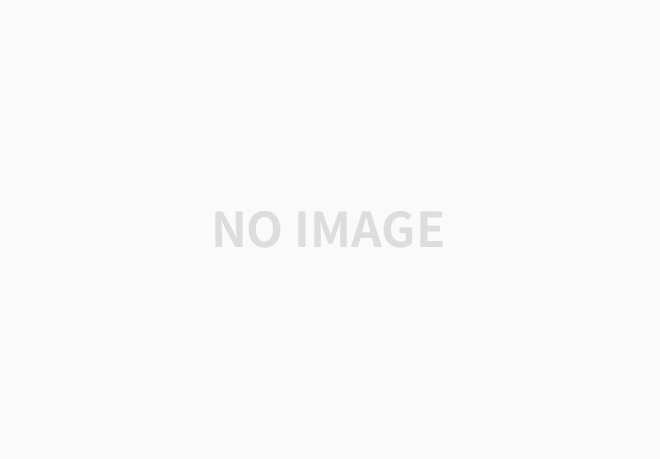
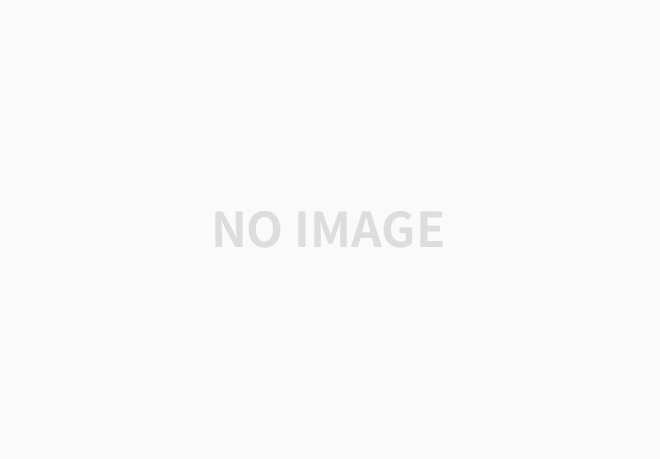
댓글